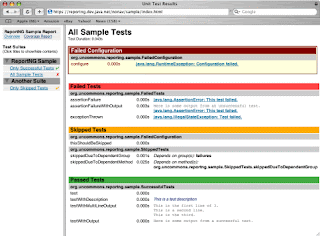
On original page there's an instruction for setup with ant, but Maven users can find this article very useful
mvn install:install-file -Dfile=sqljdbc4.jar -Dpackaging=jar -DgroupId=com.microsoft.sqlserver -DartifactId=sqljdbc4 -Dversion=4.0Then you'll get this result :
[INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building Maven Stub Project (No POM) 1 [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-install-plugin:2.4:install-file (default-cli) @ standalone-pom --- [INFO] Installing C:\sqljdbc4.jar to C:\IDEConfigs\.m2\repository\com\microsoft\ sqlserver\sqljdbc4\4.0\sqljdbc4-4.0.jar [INFO] Installing C:\Users\AF4C0~1.ZAK\AppData\Local\Temp\mvninstall797028335566 232276.pom to C:\IDEConfigs\.m2\repository\com\microsoft\sqlserver\sqljdbc4\4.0\ sqljdbc4-4.0.pom [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 0.925s [INFO] Finished at: Fri Dec 06 15:59:40 CET 2013 [INFO] Final Memory: 5M/121M [INFO] ------------------------------------------------------------------------Now you have this library in your local repository. If you want to use it in your project, then just add dependancy to your pom.xml file.
That's it. Now you can create connection to SQL Server in your testscom.microsoft.sqlserver sqljdbc4 4.0
It was a bit hard for me to get WSDL from URL, so i decided to save it locally and read it from there then. Since changes are not being made frequently in API, i can easily accept this. This configuration allows to add as many WSDL sources as i want and to store stubs for each of them in separate packages in the project. First problem was solved. Now, i had to figure out how to put my requests through Proxy . My first trial was basic and most common in Java world:org.jvnet.jax-ws-commons jaxws-maven-plugin ${jaxws.plugin.version} {put_some_id} validate wsimport {your_wsdl_location} ${project.basedir}\src\main\java\Stubs\oop ${project.basedir}\src\main\resources\wsdl\myWSDL.wsdl Stubs.oop ${project.build.directory}/jaxws/stale/wsdl.done {put_some_id} validate wsimport {your_wsdl_location} ${project.basedir}\src\main\java\Stubs\wsdl2 ${project.basedir}\src\main\resources\wsdl\your_wsld2.wsdl your_package_name ${project.build.directory}/jaxws/stale/wsdl.done
System.getProperties().put("proxySet", "true"); System.getProperties().put("proxyHost", getProxyHost()); System.getProperties().put("proxyPort", getProxyPort());But i kept getting errors and no successful connection. My next guess was that problem could be in SSL , since we are using https. So, i went into the browser, imported security certificate ( they were self-signed since it's a test environment), added them to java cacerts keystore and added next to my code :
System.setProperty("javax.net.ssl.keyStore", "keystore.jks"); System.setProperty("javax.net.ssl.trustStrore", "cacerts.jks"); System.setProperty("javax.net.ssl.keyStorePassword", "changeit");I tried to debug and to make sure that JVM is using my proxy settings and it was true - everything was there. What would be the next step in debugging for me ? I decided to install local proxy and try to put my requests through it, so i could get more details. I've installed Charles - a fantastic proxy server that helped me a lot in the past with REST API automation. It has a life changing ( for me in this case) "External Proxy" option . I've set it to forward all requests to our SOCKS proxy and then told to my tests to make calls through Charles. And it worked! You can't even believe how happy i was because of it:) But now i got another problem - Charles cost 50$ for one license:)So, there were 2 questions that i needed to solve : * Why my Java calls works fine with Charles and without it they fail? * Where to find free and easy to setup http proxy with forwarding possibility?:) With help from one of our developers we could find out why Java kept getting authentification errors and didn't want to connect to test environment properly. The problem was that java was connecting to SOCKS proxy properly, but it was resolving remote dns to another environment to which i didn't have proper certificates and credentials for authorization! I found only one article on stackoverflow regarding this issue and from that point i new that i need to have local http proxy that is : * free * can forward my requests to SOCKS proxy I've spent whole day trying to find some easy to use and adequate proxy server for Windows ( it's my Workstation that i need to use) , looked over 15 different apps and still couldn't find anything that i could use. In the end of the day i've installed VirtualBox with Ubuntu and installed there squid3 proxy server. It's the most popular and common proxy server for Unix, from what i saw on Google. But, this crazy proxy has configuration file with more then 3000 lines!! It's not that easy to make it run as i want, it's not even that easy to restart it. So, after couple hours i gave up on it and started looking for alternatives. Luckily, i found Polipo - tiny, easy to install and setup proxy server. It has few main options that i had to setup and make everything work as a charm : * proxyAddress - set it to ip address of your local virtual box, or your local machine, if you use linux or mac * allowedClients - list of ip addresses from which polipo will allow to access it and forward requests towards another proxy or web directly * socksParentProxy = "host name and port of your proxy to which i needed to forward my requests" * socksProxyType = socks5 Save changes and restart - that's it! After that i pointed my Java framework to local proxy and got green tests! To set proxy for my tests i used custom MyProxySelector class :
package Base; import java.net.*; import java.util.ArrayList; import java.util.HashMap; import java.io.IOException; public class MyProxySelector extends ProxySelector { // Keep a reference on the previous default public ProxySelector defsel = null; /** * Inner class representing a Proxy and a few extra data */ class InnerProxy { Proxy proxy; SocketAddress addr; // How many times did we fail to reach this proxy? int failedCount = 0; InnerProxy(InetSocketAddress a) { addr = a; proxy = new Proxy(Proxy.Type.HTTP, a); } SocketAddress address() { return addr; } Proxy toProxy() { return proxy; } int failed() { return ++failedCount; } } /** * A list of proxies, indexed by their address. */ HashMapAnd to turn proxy on and off i wrote next switch methods :proxies = new HashMap (); public MyProxySelector(ProxySelector def, String host, int port) { // Save the previous default defsel = def; // Populate the HashMap (List of proxies) InnerProxy i = new InnerProxy(new InetSocketAddress(host, port)); proxies.put(i.address(), i); } /** * This is the method that the handlers will call. * Returns a List of proxy. */ public java.util.List select(URI uri) { // Let's stick to the specs. if (uri == null) { throw new IllegalArgumentException("URI can't be null."); } /** * If it's a http (or https) URL, then we use our own * list. */ String protocol = uri.getScheme(); if ("http".equalsIgnoreCase(protocol) || "https".equalsIgnoreCase(protocol)) { ArrayList l = new ArrayList (); for (InnerProxy p : proxies.values()) { l.add(p.toProxy()); } return l; } /** * Not HTTP or HTTPS (could be SOCKS or FTP) * defer to the default selector. */ if (defsel != null) { return defsel.select(uri); } else { ArrayList l = new ArrayList (); l.add(Proxy.NO_PROXY); return l; } } /** * Method called by the handlers when it failed to connect * to one of the proxies returned by select(). */ public void connectFailed(URI uri, SocketAddress sa, IOException ioe) { // Let's stick to the specs again. if (uri == null || sa == null || ioe == null) { throw new IllegalArgumentException("Arguments can't be null."); } /** * Let's lookup for the proxy */ InnerProxy p = proxies.get(sa); if (p != null) { /** * It's one of ours, if it failed more than 3 times * let's remove it from the list. */ if (p.failed() >= 3) proxies.remove(sa); } else { /** * Not one of ours, let's delegate to the default. */ if (defsel != null) defsel.connectFailed(uri, sa, ioe); } } }
private ProxySelector defaultProxy = ProxySelector.getDefault(); public void setLocalProxy(){ MyProxySelector ps = new MyProxySelector(ProxySelector.getDefault(),localProxyName,localProxyPort); ProxySelector.setDefault(ps); } public void disableProxy(){ ProxySelector.setDefault(defaultProxy); }That's it. Now i can run my tests easily with control of when to use proxy and when not. In the future i'll move my tests to CI ( Jenkins most probably) and will setup on that environment Polipo proxy in 2 minutes. It's always nice to solve such non-ordinary problems. Most probably my solution is not very elegant and "right" , but it works right now and for this moment this what matters for me, since i can start writing automated tests rather then fighting with this configuration issues. *Update* . One of my colleagues proposed me to set next settings for Java :
System.setProperty("sun.net.spi.nameservice.nameservers", "ip_here"); System.setProperty("sun.net.spi.nameservice.provider.1", "dns,sun");But it didn't work for me. So will stick with my current solution for now.
yajl serialize: 0.308 deserialize: 0.334 total: 0.642 json serialize: 1.245 deserialize: 1.384 total: 2.629 cjson serialize: 0.339 deserialize: 0.244 total: 0.583 stdlib json serialize: 1.019 deserialize: 1.396 total: 2.415 ultra json serialize: 0.170 deserialize: 0.159 total: 0.328 [Finished in 7.0s]And the winner is ultra json! :) If you want to clone this script for yourself - here's the link
template = zip(*template[::-1])And an explanation for this line is next : Consider the following two-dimensional list:
original = [[1, 2], [3, 4]]Lets break it down step by step:
original[::-1] # elements of original are reversed [[3, 4], [1, 2]]This list is passed into zip() using argument unpacking, so the zip call ends up being the equivalent of this:
zip([3, 4], [1, 2]) # ^ ^----column 2 # |-------column 1 # returns [(3, 1), (4, 2)], which is a original rotated clockwiseHopefully the comments make it clear what zip does, it will group elements from each input iterable based on index, or in other words it groups the columns.
tcpdump -w output.logand then download this file on your computer, open it in Wireshark and analize with it
# tcpdump filter for HTTP GET sudo tcpdump -s 0 -A 'tcp[((tcp[12:1] & 0xf0) >> 2):4] = 0x47455420' # tcpdump filter for HTTP POST sudo tcpdump -s 0 -A 'tcp dst port 80 and (tcp[((tcp[12:1] & 0xf0) >> 2):4] = 0x504f5354)' # monitor HTTP traffic including request and response headers and message body # cf. https://sites.google.com/site/jimmyxu101/testing/use-tcpdump-to-monitor-http-traffic tcpdump -A -s 0 'tcp port 80 and (((ip[2:2] - ((ip[0]&0xf)<<2 data-blogger-escaped--="" data-blogger-escaped-tcp="" data-blogger-escaped-xf0="">>2)) != 0)' tcpdump -X -s 0 'tcp port 80 and (((ip[2:2] - ((ip[0]&0xf)<<2 data-blogger-escaped--="" data-blogger-escaped-tcp="" data-blogger-escaped-xf0="">>2)) != 0)'
tcpdump host 93.23.40.50 -s 9000 -w outputfile; perl chaosreader0.94 outputfile
The Hamming distance between two binary integers is the number of bit positions that differs (http://en.wikipedia.org/wiki/Hamming_distance). For example:
117 = 0 1 1 1 0 1 0 1 17 = 0 0 0 1 0 0 0 1 H = 0+1+1+0+0+1+0+0 = 3
def checkio(data):
a, b = data
return str(bin(a^b)).count('1')
#These "asserts" using only for self-checking and not necessary for auto-testing
if __name__ == '__main__':
assert checkio([117, 17]) == 3, "First example"
assert checkio([1, 2]) == 2, "Second example"
assert checkio([16, 15]) == 5, "Third example"
Which, we all have to admit, is cool and looks really good. For the one who just start studying python here's some short description of how this cool algorithm works :
According to python docs :
Bitwise operator works on bits and perform bit by bit operation. Assume if a = 60; and b = 13; Now in binary format they will be as follows: a = 0011 1100 b = 0000 1101 ----------------- a&b = 0000 1100 a|b = 0011 1101 a^b = 0011 0001 ~a = 1100 0011So,
nano /Applications/TorBrowser_en-US.app/Contents/MacOS/Vidalia.app/Contents/MacOS/../../../../../Library/Vidalia/torrc
ExitNodes {US}
StrictExitNodes 1
Save it, stop Tor and then start it again
Now go to https://play.google.com/store and you'll see that devices section is available for you and you can actually select and purchase one of them:)
python server.py 8081 0&